In this section, we’ll explore techniques for centering images in Bootstrap 5. There are various methods available, including the use of flexbox, grid, and more.
Starting Bootstrap 5 Structure with CDN
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>Bootstrap 5 image center</title>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN"
crossorigin="anonymous"
/>
</head>
<body>
<!-- content -->
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL"
crossorigin="anonymous"
></script>
</body>
</html>
Certainly! Apply the Bootstrap 5 text-center
class to a container element, and enclose your image within a div to achieve image centering.
<div class="text-center">
<img src="https://picsum.photos/200.jpg" class="img-fluid" alt="Your Image">
</div>
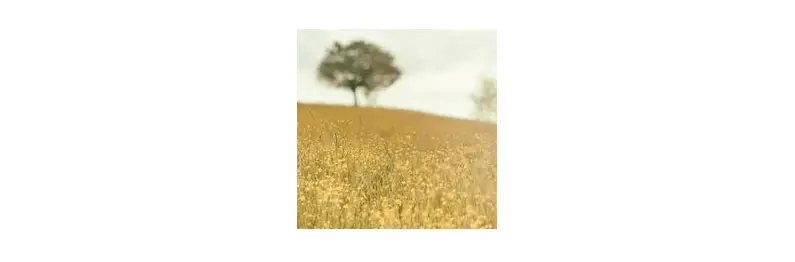
Using Flexbox utility classes
For greater control, especially in complex layouts, utilize Flexbox utilities. Place your image within a container div and apply d-flex
and justify-content-center
classes.
<div class="d-flex justify-content-center">
<img src="https://picsum.photos/200.jpg" class="img-fluid" alt="Your Image">
</div>
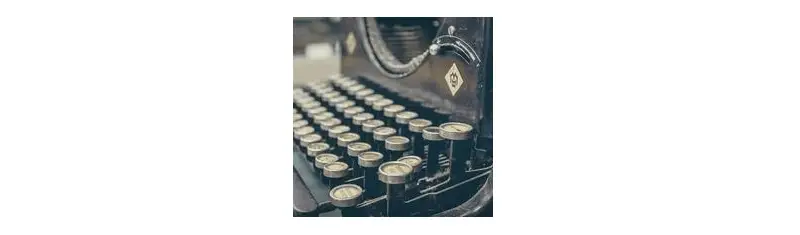
Centering vertically and horizontally
To centrally align an image both vertically and horizontally, employ Flexbox utilities. Use d-flex, justify-content-center, and align-items-center on the container, ensuring it has a specified height.
<div class="d-flex justify-content-center align-items-center" style="height: 300px;">
<img src="https://picsum.photos/200.jpg" class="img-fluid" alt="Your Image">
</div>
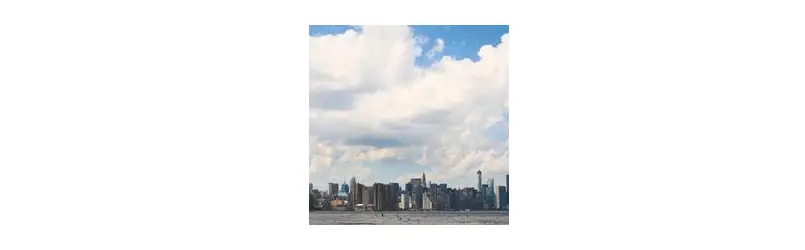
Centering Using Grid System
Bootstrap’s grid system can also be used to center images, especially when you want to position the image in a specific column layout.
<div class="container">
<div class="row justify-content-center">
<div class="col-6">
<img src="path/to/your/image.jpg" class="img-fluid" alt="Your Image">
</div>
</div>
</div>
Centering with Margin Auto
You can center an image by applying auto margins. This is useful when you want a simple centering without affecting the text alignment or using Flexbox/grid layout.
<div style="width: 100%; display: block;">
<img src="path/to/your/image.jpg" class="mx-auto d-block img-fluid" alt="Your Image">
</div>