CSS selectors have the ability to target HTML elements by utilizing different attributes, including custom data attributes. Custom data attributes are attributes that begin with data- followed by a name of your choosing. These attributes are typically used to store additional information about HTML elements. Their purpose is often to facilitate JavaScript interactions or for styling purposes. To select elements based on data attributes in CSS, here’s what you need to do:
Attribute Selectors:
You can target elements based on their data attributes using attribute selectors.
[element][data-attribute="value"] {
/* styles */
}
Wrap the HTML element you wish to select (e.g., div, span, input, etc.) within square brackets along with the specific data attribute name preceded by data- and specify the desired value inside quotation marks. For instance, [data-attribute=”value”].
Multiple Attribute Selectors:
Replace the targeted HTML element, data attribute, and value. You can combine multiple attributes, including data attributes, to target elements more precisely:
[element][data-attribute1="value1"][data-attribute2="value2"] {
/* styles */
}
Partial Value Matching:
You can target elements based on partial attribute values using the ^, $, *, | symbols: ^ for starts with, $ for ends with, * for contains, | for matches a hyphen-separated list.
/* Selects all elements with a data-attribute that starts with 'prefix-' */
[data-attribute^="prefix-"] {
/* styles */
}
/* Selects all elements with a data-attribute that ends with '-suffix' */
[data-attribute$="-suffix"] {
/* styles */
}
/* Selects all elements with a data-attribute that contains 'substring' */
[data-attribute*="substring"] {
/* styles */
}
/* Selects all elements with a data-attribute that exactly matches 'lang' or begins with 'lang-' */
[data-attribute|="lang"] {
/* styles */
}
Custom Styling for Error Messages
<div class="error-message" data-type="warning">Warning: Please fill in all fields.</div>
<div class="error-message" data-type="error">Error: Passwords do not match.</div>
<div class="error-message" data-type="info">Info: Your session will expire soon.</div>
/* Styling error messages */
.error-message {
padding: 10px;
margin-bottom: 10px;
}
/* Custom styling based on data attribute */
[data-type="warning"] {
color: #ff6600; /* orange */
}
[data-type="error"] {
color: #ff0000; /* red */
}
[data-type="info"] {
color: #3366ff; /* blue */
}
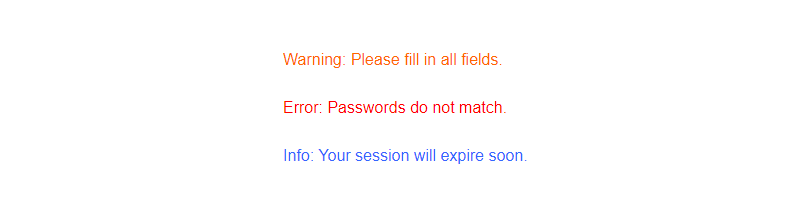
Customizing Form Inputs
<input type="text" placeholder="Username" data-input="username">
<input type="password" placeholder="Password" data-input="password">
<input type="email" placeholder="Email" data-input="email">
/* Styling form inputs */
input[type="text"],
input[type="password"],
input[type="email"] {
width: 200px;
padding: 5px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
/* Custom styling based on data attribute */
[data-input="username"] {
background-color: #f0f0f0; /* light grey */
}
[data-input="password"] {
background-color: #ffe6e6; /* light red */
}
[data-input="email"] {
background-color: #e6f9ff; /* light blue */
}
